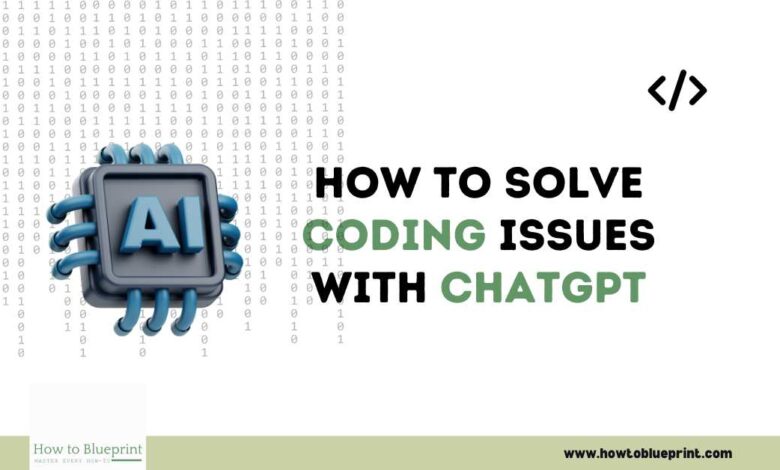
In the fast-paced world of software development, encountering coding issues is an inevitable part of the journey. From syntax errors to complex logic bugs, resolving these challenges swiftly and effectively is crucial for maintaining productivity and ensuring project success. With advancements in artificial intelligence, particularly models like OpenAI‘s ChatGPT, developers now have a powerful ally to assist in navigating these coding hurdles. This article explores in depth how to leverage ChatGPT to effectively solve coding issues, providing actionable insights, practical examples, and best practices. Solve Coding Issues with ChatGPT.
Understanding ChatGPT
What is ChatGPT?
ChatGPT, based on the advanced GPT-4 architecture developed by OpenAI, is a state-of-the-art language model trained on a vast corpus of text data. It excels in understanding and generating human-like text responses. Unlike traditional search engines or static documentation, ChatGPT offers interactive, context-aware assistance, making it an invaluable tool for developers tackling coding challenges.
Capabilities and Limitations
Capabilities of ChatGPT
- Code Debugging: ChatGPT can identify and suggest corrections for syntax errors and logic bugs, helping developers troubleshoot their code effectively.
- Code Generation: It can generate code snippets based on natural language descriptions, facilitating rapid prototyping and exploration of new ideas.
- Concept Explanation: ChatGPT can explain complex programming concepts in simple terms, making it an excellent resource for both beginners and experienced developers.
- Code Review: It can review code for potential issues, suggest optimizations, and ensure adherence to best practices.
- Versatility: ChatGPT supports multiple programming languages and frameworks, providing versatile assistance across various technology stacks.
Limitations to Consider
- Contextual Understanding: While ChatGPT can maintain context within a session, it may struggle with long-term memory and maintaining context across extended interactions.
- Knowledge Cutoff: The model’s knowledge is based on data up to its training cutoff date (September 2021 for GPT-4), potentially lacking awareness of the latest updates or technologies beyond that point.
- Ambiguity Handling: Vague or ambiguous queries may lead to incomplete or incorrect responses. Clear and specific questions yield more accurate results.
- Real-time Execution: ChatGPT cannot execute code in real-time, limiting its ability to dynamically test or debug live applications.
Getting Started with ChatGPT for Coding Issues
Accessing ChatGPT
ChatGPT is accessible through various platforms, including OpenAI’s official website, integrated development environments (IDEs), and third-party applications. Popular integrations include:
- OpenAI Playground: A web-based interface for interacting with GPT-4.
- IDE Extensions: Plugins for editors like Visual Studio Code.
- Chat Platforms: Integrations with Slack and Discord for collaborative coding environments.
Setting Up Your Environment
Before utilizing ChatGPT for coding assistance, ensure your development environment is prepared:
- Tool Installation: Install necessary programming language compilers, interpreters, and relevant libraries.
- IDE Integration: Integrate ChatGPT into your preferred IDE for seamless interaction and productivity.
- Resource Accessibility: Keep documentation and reference materials readily accessible for quick consultation during coding sessions.
Solve Coding Issues with ChatGPT
Identifying and Addressing Coding Problems
Syntax Errors
Syntax errors are fundamental issues violating the grammar rules of a programming language. Common errors include missing semicolons, mismatched parentheses, and incorrect indentation.
Example:
def greet(name)
print("Hello, " + name)
ChatGPT Solution:
ChatGPT identifies the missing colon after the function definition and suggests the correct syntax:
def greet(name):
print("Hello, " + name)
Logic Errors
Logic errors occur when code executes without syntax issues but produces unintended results due to flawed algorithms or incorrect assumptions.
Example:
def calculate_area(length, width):
return length + width
# Expected output is the product of length and width, not the sum.
ChatGPT Solution:
ChatGPT analyzes the logic and proposes the correct formula for calculating the area:
def calculate_area(length, width):
return length * width
Runtime Errors
Runtime errors occur during code execution and can result from operations like dividing by zero or accessing out-of-bounds array indices.
Example:
def divide(a, b):
return a / b
# Calling divide(10, 0) will raise a ZeroDivisionError.
ChatGPT Solution:
ChatGPT recommends implementing error handling to prevent runtime errors:
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
return "Error: Division by zero is not allowed."
Debugging Techniques with ChatGPT
Step-by-Step Debugging Assistance
ChatGPT guides developers through systematic debugging processes, helping isolate and resolve issues methodically.
Example:
def find_max(numbers):
max_number = numbers[0]
for number in numbers:
if number > max_number:
max_number = number
return max_number
Debugging Steps with ChatGPT:
- Initialization Verification: Ensure
max_number
is initialized correctly. - Loop Inspection: Confirm the loop iterates over all elements.
- Condition Validation: Validate the condition for updating
max_number
.
ChatGPT confirms the code correctness and may suggest handling edge cases:
def find_max(numbers):
if not numbers:
return None # Handle empty list case
max_number = numbers[0]
for number in numbers:
if number > max_number:
max_number = number
return max_number
Automated Testing Support
Effective testing is essential for code reliability. ChatGPT can aid in generating test cases and enhancing test coverage.
Example:
import unittest
class TestMathFunctions(unittest.TestCase):
def test_calculate_area(self):
self.assertEqual(calculate_area(5, 10), 50)
self.assertEqual(calculate_area(0, 10), 0)
self.assertEqual(calculate_area(-5, 10), -50)
if __name__ == '__main__':
unittest.main()
ChatGPT suggests additional test cases and edge scenarios to improve test coverage.
Generating Code with ChatGPT
From Description to Code Implementation
ChatGPT excels in transforming natural language descriptions into functional code snippets, accelerating development tasks like boilerplate code creation.
Example:
Description: Create a function that filters even numbers from a list and returns a new list.
ChatGPT Solution:
def filter_even_numbers(numbers):
return [number for number in numbers if number % 2 == 0]
Refactoring and Optimization Guidance
Improving code readability and performance is another area where ChatGPT proves invaluable, offering suggestions for refactoring and optimization.
Example:
Original Code:
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
ChatGPT Refactored Code:
def process_data(data): return [item * 2 for item in data if item % 2 == 0]
Learning and Exploring New Concepts
Concept Explanation
ChatGPT serves as an educational resource, providing clear explanations of programming concepts and principles.
Example:
Question: What is a closure in Python?
ChatGPT Answer:
A closure in Python is a function object that retains access to variables in its lexical scope, even when the function is called outside that scope. Closures are created when a nested function references a value from its enclosing scope.
Example:
def outer_function(message):
def inner_function():
print(message)
return inner_function
closure = outer_function("Hello, World!")
closure() # Outputs: Hello, World!
Introduction to New Libraries and Frameworks
When exploring unfamiliar libraries or frameworks, ChatGPT provides introductory guidance and practical examples.
Example:
Question: How do I use the requests
library in Python to make a GET request?
ChatGPT Answer:
The requests
library simplifies HTTP requests in Python. To make a GET request, use the following code snippet:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
print(response.json())
else:
print(f"Error: {response.status_code}")
Code Review and Best Practices
Identifying and Addressing Issues
ChatGPT assists in code reviews by highlighting potential issues such as performance bottlenecks, security vulnerabilities, and adherence to coding best practices.
Example:
Original Code:
def read_file(file_path):
file = open(file_path, 'r')
data = file.read()
file.close()
return data
ChatGPT Review:
- Resource Management: Recommends using a context manager to ensure proper file handling.
- Error Handling: Suggests incorporating error handling for file operations.
Refactored Code:
def read_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read()
except IOError as e:
print(f"Error reading file: {e}")
return None
Promoting Coding Best Practices
ChatGPT offers insights into coding best practices, ensuring code is
clean, efficient, and maintainable.
Example:
Question: What are some best practices for writing Python functions?
ChatGPT Answer:
- Modularity: Aim for functions that perform a single, well-defined task.
- Descriptive Naming: Use clear and meaningful names for functions and variables.
- Documentation: Include docstrings to describe parameters, return values, and function behavior.
- Error Handling: Implement robust error handling to manage unexpected scenarios.
- Avoid Global Variables: Minimize the use of global variables to enhance code clarity and maintainability.
Advanced Usage Scenarios
Integrating ChatGPT into Applications
Building Chatbots and Interactive Interfaces
ChatGPT can be integrated into chatbots and interactive applications to provide real-time coding assistance and support.
Example:
Using a chatbot interface powered by ChatGPT, developers can ask coding-related questions and receive immediate feedback, examples, and explanations.
Enhancing Collaborative Development
Pair Programming Support
Pair programming involves two developers working together at one workstation. ChatGPT can act as a virtual pair programmer, offering suggestions, identifying issues, and providing alternative solutions in real-time.
Example:
During a pair programming session, ChatGPT can assist one developer by suggesting improvements or spotting potential errors as code is being written.
Automating Documentation
Code Documentation Automation
Maintaining comprehensive documentation is essential but time-consuming. ChatGPT can automate the documentation process by generating docstrings, comments, and detailed documentation from the codebase.
Example:
Given a function, ChatGPT can generate a detailed docstring:
def add(a, b):
return a + b
# ChatGPT Generated Docstring
"""
Adds two numbers and returns the result.
Parameters:
a (int, float): The first number to add.
b (int, float): The second number to add.
Returns:
int, float: The sum of the two numbers.
"""
Supporting Continuous Learning
Learning and Skill Development
ChatGPT serves as a personalized tutor, helping developers learn new programming languages, frameworks, and tools through explanations, examples, and interactive exercises.
Example:
A developer interested in learning JavaScript can engage with ChatGPT to receive tutorials starting from foundational concepts to advanced topics, accompanied by code demonstrations.
Best Practices for Using ChatGPT
Crafting Effective Queries
To optimize interactions with ChatGPT, formulate clear and specific queries. Avoid ambiguity to receive accurate and actionable responses.
Example:
Instead of asking, “How do I fix this code?”, specify the issue: “Why does this Python function return None instead of the expected result?”
Iterative Refinement
Iteratively refine queries if initial responses are not satisfactory. Provide additional details or ask follow-up questions to narrow down the issue effectively.
Example:
First Query: “How can I optimize this code?” Refined Query: “What strategies can I employ to reduce the time complexity of this sorting algorithm?”
Leveraging Additional Resources
While ChatGPT is a valuable tool, complement its insights with other resources such as official documentation, forums, and tutorials to deepen understanding and enhance problem-solving.
Example:
Use ChatGPT for initial guidance on a new library, then consult official documentation for comprehensive details and advanced usage scenarios.
Security Considerations
When sharing code or discussing project details with ChatGPT, avoid including sensitive information like passwords, API keys, or proprietary code. Exercise caution similar to any online platform interaction.
Validating Suggestions
Validate ChatGPT’s suggestions by testing and reviewing in your development environment. Ensure solutions align with project requirements, coding standards, and performance expectations.
Example:
After receiving a code suggestion, thoroughly test it in your environment to verify correctness and assess performance impact.
Conclusion
ChatGPT represents a significant advancement in AI-driven coding assistance, offering developers a versatile tool for tackling coding challenges across various stages of software development. By harnessing ChatGPT’s capabilities to identify and resolve coding issues, generate code snippets, explain concepts, and promote best practices, developers can streamline workflows, improve code quality, and accelerate project timelines.
As AI technology continues to evolve, tools like ChatGPT will play an increasingly integral role in enhancing developer productivity, supporting continuous learning, and fostering innovation in software development. By mastering the art of leveraging ChatGPT effectively and integrating it into their development workflows, developers can stay ahead in the ever-changing landscape of technology and coding practice
One Comment