How to Create Custom Widgets in WordPress
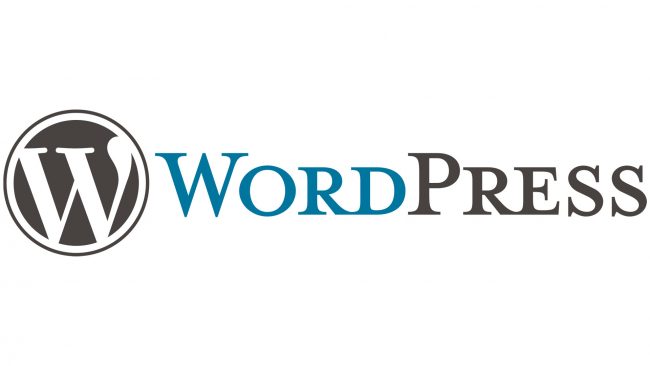
Think of your WordPress website as a blank canvas. Widgets are the versatile tools that let you paint on dynamic elements – anything from a simple list of recent posts to a complex social media feed. But what if the default widgets don’t quite cut it? Enter custom widgets – your secret weapon for crafting a website that’s truly one-of-a-kind.
In this guide, we’ll walk you through the process of creating your very own custom widgets, step by step. Whether you’re a beginner coder or a seasoned WordPress pro, you’ll discover how to add unique functionality and personalization to your site.
Why Custom Widgets Are a Game-Changer
- Tailored to Your Vision: Default widgets are generic. Custom widgets let you build features that match your website’s specific needs and aesthetics.
- Enhanced Functionality: Want to display a countdown timer, a custom contact form, or even a mini-game? Custom widgets can make it happen.
- Streamlined User Experience: Widgets can be placed in sidebars, footers, or even within your content, making it easy for visitors to access key information or interact with your site.
Real-World Fact: Over 50% of the top 1 million websites use WordPress. This means countless websites are using custom widgets to stand out from the crowd!
Understanding the Building Blocks: Widgets 101
Before we dive into the coding, let’s cover the basics:
- What is a widget? A widget is a self-contained block of code that displays specific content or functionality on your website.
- Where do they live? Widgets are typically placed in designated widget areas (like sidebars or footers) defined by your theme.
- How do they work? WordPress provides a Widget API (Application Programming Interface) that allows you to create and register custom widgets.
Your Toolkit: Essential Tools for Custom Widget Creation
- Code Editor: You’ll need a text editor to write your widget code. Popular choices include Visual Studio Code, Sublime Text, or Atom.
- WordPress Installation: You’ll need a working WordPress site to test and implement your custom widgets.
- Basic PHP Knowledge: While you don’t need to be a PHP expert, a basic understanding of the language will help you grasp the concepts involved.
The Step-by-Step Guide to Crafting Your First Custom Widget
-
Create a New PHP File: In your theme’s directory, create a new PHP file (e.g.,
custom-widget.php
). This is where you’ll write your widget code. -
Define Your Widget Class: Use the following code structure as a template:
class My_Custom_Widget extends WP_Widget { // Constructor function __construct() { // Widget details (name, description, etc.) } // Output the widget's content on the front-end public function widget( $args, $instance ) { // Your custom code to display the widget content } // Output the widget's settings form in the admin area public function form( $instance ) { // Fields for users to customize the widget } // Save the widget's settings public function update( $new_instance, $old_instance ) { // Process and save the widget settings } }
-
Register Your Widget: Add the following code to your theme’s
functions.php
file to register the widget with WordPress:function register_my_custom_widget() { register_widget( 'My_Custom_Widget' ); } add_action( 'widgets_init', 'register_my_custom_widget' );
-
Customize Your Widget: Fill in the
widget()
,form()
, andupdate()
functions with your own code to define the widget’s content, settings, and behavior.
Real-World Example: Creating a “Recent Posts with Thumbnails” Widget
Let’s say you want a widget that displays your latest blog posts with their featured images. Here’s a simplified example of how you might implement it:
// ... (rest of the class code)
public function widget( $args, $instance ) {
echo $args['before_widget'];
$query_args = array(
'posts_per_page' => 5, // Number of posts to display
'post_type' => 'post'
);
$recent_posts = new WP_Query( $query_args );
if ( $recent_posts->have_posts() ) {
echo '<ul>';
while ( $recent_posts->have_posts() ) {
$recent_posts->the_post();
echo '<li>';
if ( has_post_thumbnail() ) {
the_post_thumbnail( 'thumbnail' ); // Display thumbnail
}
echo '<a href="' . get_permalink() . '">' . get_the_title() . '</a>';
echo '</li>';
}
echo '</ul>';
}
wp_reset_postdata(); // Reset the query
echo $args['after_widget'];
}
Tips and Tricks for Widget Wizardry
- Enqueue Styles and Scripts: If your widget uses custom CSS or JavaScript, make sure to enqueue them properly to avoid conflicts.
- Use Filters and Actions: WordPress provides hooks (filters and actions) that allow you to modify the behavior of your widgets or integrate them with other plugins.
- Test Thoroughly: Always test your custom widgets on different browsers and devices to ensure they work as expected.
Troubleshooting Common Widget Woes
- Widget Not Appearing: Double-check that you’ve registered the widget correctly and that your theme has a widget area where you can place it.
- Error Messages: If you encounter PHP errors, carefully review your code for typos or syntax issues.
- Plugin Conflicts: If your widget doesn’t work properly with certain plugins, try deactivating those plugins temporarily to see if they are causing the conflict.
Conclusion: Your Website, Your Way
With the power of custom widgets at your disposal, you can transform your WordPress site into a dynamic and personalized platform that truly reflects your brand or message. Don’t be afraid to experiment and explore the possibilities – the only limit is your imagination!
One Comment